chrome download shelf
Chrome download shelf is the widget shown in the buttom when you initiate a new download. This document summarizes how the download shelf is implemented. It is sketchy on the details. For more information, click on the source code or documentation links.
How to inspect chrome browser ui components
In short, run chrome with chromium --enable-ui-devtools=1234
and open url chrome://inspect/#native-ui
.
See also UI DevTools Overview and Chromium Desktop UI Debugging Tools and Tips.
Layout in browser window
How to layout a new component
The elements of chromium UI are views. A View is a UI element, similar to an HTML DOM element. In order to create new UI componenets, we need to create corresponding views.
Take download shelf as an example, the entrypoint to register new browser views is browser_view_layout.cc
.
Here is how chromium layout download shelf in the main window, where the download_shelf_
is a view created here.
Download shelf
Implementations
There are currently two implementations for the download shelf. One is implemented in c++ with MVC architecture, while the other is implemented with webui using web technologies.
MVC Architecture
Chromium uses the well-known MVC design pattern to layout UI components, although sometimes model, view and controller are not strictly separated.
Here is a diagram which illustrates how mvc architecture works.
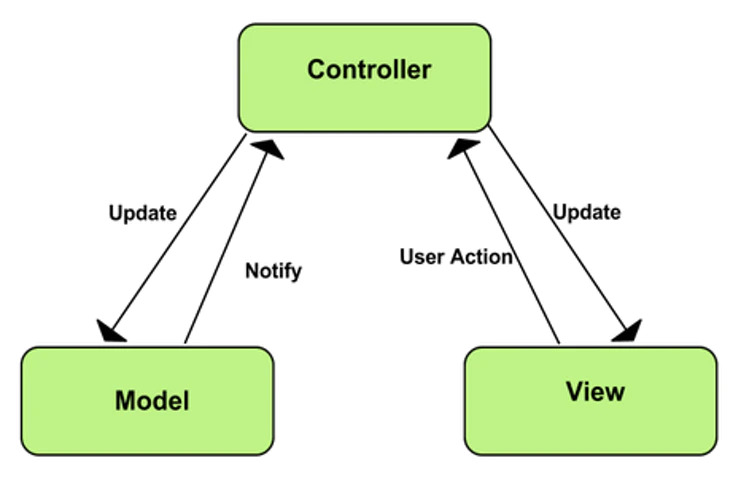
The download shelf view is the UI that is visiable to the user. The user may interact with the download shelf, which then leverages the controller functions to update the download items.
For example, when the user click on the context menu (shown by DownloadShelfWebView::ShowDownloadContextMenu
) of a download item on the download shelf. The download shelf view executes this download command by
calling DownloadCommands::ExecuteCommand
, which in turn calls DownloadUIModel::ExecuteCommand of the DownloadUIModel
.
When the download items are modified, the views may be updated according by the controllers. The download item models notify controller for updates using the observer design pattern.
For example, DownloadUIController
observes download item modifications, the notifications are sent by the download manager core services (DownloadNotificationManager
).
When a new download item is ready, download core service calls DownloadNotificationManager::OnNewDownloadReady
, which then calls DownloadShelfUIControllerDelegate::OnNewDownloadReady
, which in turn
calls browser->window()->GetDownloadShelf()->AddDownload(std::move(model))
to make the download item show up on the download shelf.
Download items
There are three data models for the download items.
Download UI model
DownloadUIModel
is an interface to define methods to operate on download items (e.g. pasue, resume downloads), show download item status (e.g. saved file name, downloading status).
Download item model
DownloadItemModel
is just a wrapper around download::DownloadItem
, which implements the download UI model interface.
Download item
download::DownloadItem
is the underlying download item implementation. It defines what represents a download item, how to serialize download items, how to store their states, etc.
Menu and commands
To show a simple context menu, we need to inherit ui::SimpleMenuModel::Delegate
. A SimpleMenuModel::Delegate
subclass needs to define some commands and their actions.
Commands correspond to menu items. When a menu item is clicked, the corresponding action is executed.
Webui download shelf
“WebUI” is a term used to loosely describe parts of Chrome’s UI implemented with web technologies (i.e. HTML, CSS, JavaScript).
Communication Between the Browser and WebUI web page
Bi-direction communication by mojo (a high level IPC abstraction).
Data definition and RPC
The data structures and rpc interfaces are defined in download_shelf.mojom
. It contains what structures represent a download when passing from browser to webpage, and vice versa, and what remote procedure calling does the browser provide.
Note that the download items defined here is different from download::DownloadItem
. We may need to convert from one to another.
Browser side handler
Browser side handlers are normally called PageHandler
. The download shelf PageHandler
defines methods to accept rpc request from the website, execute the relevant methods, and return the results.
Examples are opening the downloaded item, removing a download. The interface is here. It is implemented here in the browser. The method calls are delegated to the underlying download models.
The webui client calls these methods by the generated mojo apis.
Web page side handler
Web page side handlers are normally called Handler
. They are used by the web page to handled native RPC requests from the browser. When the browser did something, it may want to notify the browser for its effects,
e.g. there is a new download, the webui may want to show it on its interface. Here are the download shelf web page handlers. There are implmented in javascript here.
UI embedding
The webui have low barriers to entry, but it may lack some desirable effects. For example, we may need to show a native menu. We need to define a UI embedder to show native context menu.
The download shelf define a DownloadShelfUIEmbedder
here. When the user right clicks on a download item, he actually sees the context menu of the DownloadShelfContextMenuView
which is able to display native context menu.
WebUI registration
To register a chrome://
url for a webui, we need to register it in the GetWebUIFactoryFunction
. Here is how download shelf is registered.